What is Rest API in java
Understanding REST API
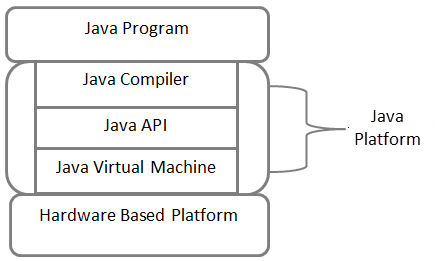
Before diving into REST API in Java, let’s first understand what REST API is in general. REST is an architectural style that defines a set of constraints for building web services. It is based on the principles of simplicity, scalability, and statelessness. REST APIs use the HTTP protocol for communication and leverage its various methods such as GET, POST, PUT, and DELETE to perform different operations on resources.
In the context of Java programming, REST API refers to the implementation of RESTful web services using Java technologies. Java provides a robust ecosystem for building REST APIs, with frameworks like JAX-RS (Java API for RESTful Web Services) facilitating the development process. These frameworks provide a set of classes, interfaces, and annotations that simplify the creation and deployment of RESTful web services.
Benefits of REST API in Java
REST API in Java offers several advantages that make it a popular choice for building web services. Here are some of the key benefits:
1. Simplicity and Lightweight
REST API is known for its simplicity and lightweight nature. It follows a resource-based approach, where each resource is identified by a unique URI (Uniform Resource Identifier). This simplicity makes it easier to understand, implement, and maintain RESTful web services in Java.
2. Scalability and Performance
REST API’s stateless nature allows it to scale easily. Each request to a RESTful web service is independent of previous requests, making it suitable for distributed and scalable systems. Additionally, REST API’s use of HTTP caching mechanisms enhances performance by reducing server load and network latency.
3. Platform-Independent
REST API in Java is platform-independent, meaning it can be consumed by clients built on different programming languages and frameworks. This interoperability makes it highly versatile and enables easy integration with existing systems.
4. Flexibility and Modularity
REST API promotes a modular and flexible design by separating the client and server concerns. This separation allows independent evolution and updates of the client and server components. Developers can easily add, modify, or remove resources without impacting the overall system.
5. Wide Industry Adoption
REST API has gained widespread adoption in the industry, making it a standard choice for building web services. Many popular platforms and services, such as Twitter, Facebook, and Google, expose their functionality through RESTful APIs. This popularity ensures a large community of developers and extensive documentation and support.
Working with REST API in Java
To work with REST API in Java, developers can leverage frameworks like JAX-RS, which provide a set of annotations and classes to simplify the development process. JAX-RS allows developers to define RESTful resources using annotations, map them to URI paths, and specify the HTTP methods for each resource.
Let’s take a look at a real-world example of working with REST API in Java using the JAX-RS framework. Suppose we want to build a simple RESTful web service that exposes an endpoint for retrieving user information.
First, we need to define a resource class that represents the user resource. We can use JAX-RS annotations to specify the URI path and HTTP method for this resource:
@Path("/users")
public class UserResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public User getUser() {
User user = new User("John Doe", "john.doe@example.com");
return user;
}
}
In the above example, the @Path
annotation specifies that this resource is mapped to the /users
path. The @GET
annotation indicates that this method should handle GET requests. The @Produces
annotation specifies the media type of the response, which in this case is JSON.
To deploy this RESTful web service, we need to configure a servlet container like Apache Tomcat and package the application as a WAR (Web Application Archive) file. Once deployed, the user information can be accessed by making a GET request to the /users
endpoint.
Required Skills for Working with REST API in Java
To effectively work with REST API in Java, it is essential to have a solid understanding of the following skills:
1. Knowledge of MVC Frameworks
Knowledge of MVC (Model-View-Controller) frameworks like Spring or Play is essential for building RESTful web services in Java. These frameworks provide a structured approach to handle the separation of concerns and facilitate the development of scalable and maintainable applications.
2. Understanding of HTTP Protocol
A thorough understanding of the HTTP protocol is crucial when working with REST API in Java. It is important to be familiar with the various HTTP methods (GET, POST, PUT, DELETE) and their corresponding semantics. Additionally, understanding HTTP headers, status codes, and caching mechanisms is essential for designing robust and efficient RESTful web services.
3. Knowledge of Data Formats
REST API in Java often involves working with different data formats like JSON or XML. It is important to have a good understanding of these formats and how to parse and serialize data in these formats. Additionally, familiarity with technologies like JSON or XML parsers is beneficial when working with RESTful web services.
4. Basics of Validation Framework
Validating incoming data is an important aspect of building secure and reliable RESTful web services. Having knowledge of a validation framework, such as Hibernate Validator or Spring Validation, allows developers to enforce data integrity and ensure the correctness of data consumed or produced by the API.
5. Familiarity with Persistence Systems
RESTful web services often interact with databases or other data stores to retrieve or store data. Having knowledge of persistence systems like Spring Data or Hibernate enables developers to efficiently manage data persistence and perform CRUD (Create, Read, Update, Delete) operations.
Fundamentals of REST API in Java
To truly master REST API in Java, it is important to have a good grasp of the following fundamentals:
1. Building a Basic REST API
Start by building a basic REST API that responds with a simple message or web content. This will help you understand the fundamental concepts of REST and how to handle different HTTP methods.
2. Consuming and Producing JSON/XML
Learn how to consume and produce data in JSON or XML formats. This involves parsing incoming data, validating it if necessary, and serializing data to the desired format for the response.
3. Handling Form Submissions
Understand how to handle form submissions in RESTful web services. This includes receiving form data, validating it, and storing it in a database or other data store.
4. Connecting to Other APIs
Learn how to connect to other APIs and consume their data in your REST API. This involves making HTTP requests to external APIs, handling the responses, and processing the data accordingly.
5. Persisting Data to Data Stores
Understand how to persist data to various data stores, both SQL and NoSQL. This involves mapping Java objects to database tables or document collections and performing CRUD operations using appropriate persistence frameworks.
6. Updating and Deleting Data
Learn how to update and delete data in a database through your REST API. This involves implementing the necessary endpoints and handling the corresponding HTTP methods.
7. Securing Your API
Understand the importance of securing your REST API to protect sensitive data and prevent unauthorized access. This includes implementing authentication and authorization mechanisms, such as token-based authentication or OAuth.
By mastering these fundamentals, you can become a proficient REST API developer in Java.
Why Do We Need REST API in Java?
REST API in Java serves various purposes and provides significant benefits. Here are some reasons why we need REST API in Java:
1. Streamlining Techniques
REST API allows us to streamline techniques by consolidating multiple actions into a single view. For example, social media platforms like Facebook and Twitter utilize REST APIs to provide users with a unified inbox, where they can view and respond to messages from different platforms in one place.
2. Making Applications Easier
REST API simplifies application development by providing access to various software components. This flexibility enables developers to deliver services and data more efficiently, resulting in improved user experiences.
3. Business Expansion
By providing an API, businesses can extend their reach and offerings to a wider audience. APIs enable developers to integrate a company’s services and resources into their own applications, allowing for additional customers and increased revenue opportunities.
How REST API in Java Can Help in Career Growth
Proficiency in REST API development in Java can significantly contribute to career growth. Many multinational corporations, such as Flipkart, Walmart, Amazon, and Goldman Sachs, rely on Java API for their large-scale projects. Java API developers are in high demand due to the stability, scalability, and object-oriented nature of Java programming. With Java API expertise, developers can find opportunities in various domains, including Android applications, web applications, big data technologies, payments, e-commerce, and more.
Conclusion
REST API in Java offers a powerful and versatile way to build web services. Its simplicity, scalability, and platform-independent nature make it a popular choice among developers. By mastering the required skills and understanding the fundamentals, developers can leverage REST API in Java to create robust and efficient web services. With the increasing demand for REST API developers in the industry, learning and mastering REST API in Java can pave the way for career growth and exciting opportunities. So, start exploring the world of REST API in Java and unlock its potential for your projects and career.
Recommended Articles
To further enhance your understanding of REST API in Java, check out these recommended articles:
- Introduction to MVC Design Pattern
- HTTP Methods and Their Usage
- JSON vs XML: A Comparison
- Advantages of Object-Oriented Programming
- Big Data Technologies and Their Applications
Remember, continuous learning and hands-on practice are key to becoming a proficient REST API developer in Java. So, keep exploring, experimenting, and expanding your knowledge in this exciting field.

Business Analyst , Functional Consultant, Provide Training on Business Analysis and SDLC Methodologies.